Elite Hoops Year One: 12 Bite-Sized Lessons
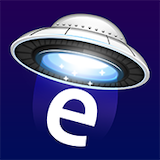
This post is brought to you by Emerge Tools, the best way to build on mobile.
Elite Hoops has hit the one year mark! I had a few buddies ask me some questions around getting those first paying customers, the kinds of marketing I’ve tried and other similar things.
In short, indies talkin’ shop. So, here is a point-by-point brain dump of what I think the twelve most helpful things I’ve learned or tried are. I’ll try to keep each one around a few sentences to keep it to the point. But, you know, IChat(.aLot)
.
In you just want the TL;DR on the #numbies, here’s that:
- 860 paying customers
- $3,000 MRR
Cool, on to the good stuff.
3 Tips on Getting the First 100 Paying Users
On getting to that 100 paying user mark, which was my first goal.
1: Ask for Emails in Exchange for ✨Value✨
Setup an email capture component. A form, use an API, whatever. This is a fantastic way to let people know of large updates, push sales and reactivate folks. I use Mailerlite, and it’s okay, but adding an option to join the mailing list during onboarding to learn about plays, free stuff and more means that a little over 30% of people join it.
let concatenatedThoughts = """
Also, use the right text content types on your sign up fields so iOS will offer to autofill their email.
"""
2: Scaling with Paid Ads
I spend $30 in Meta ads every single day1. It took about three months, but I found a winning creative and audience after experimenting. I think a lot of indies either say paid acquisition is “lighting money on fire” or they take too much pride in touting “organic growth” at the expense of, well — more growth. You don’t have to spend a ton of money, you can get meaningful results with a little money.
Here is an example of the ad I run most. I make all of the creatives in Sketch, too:
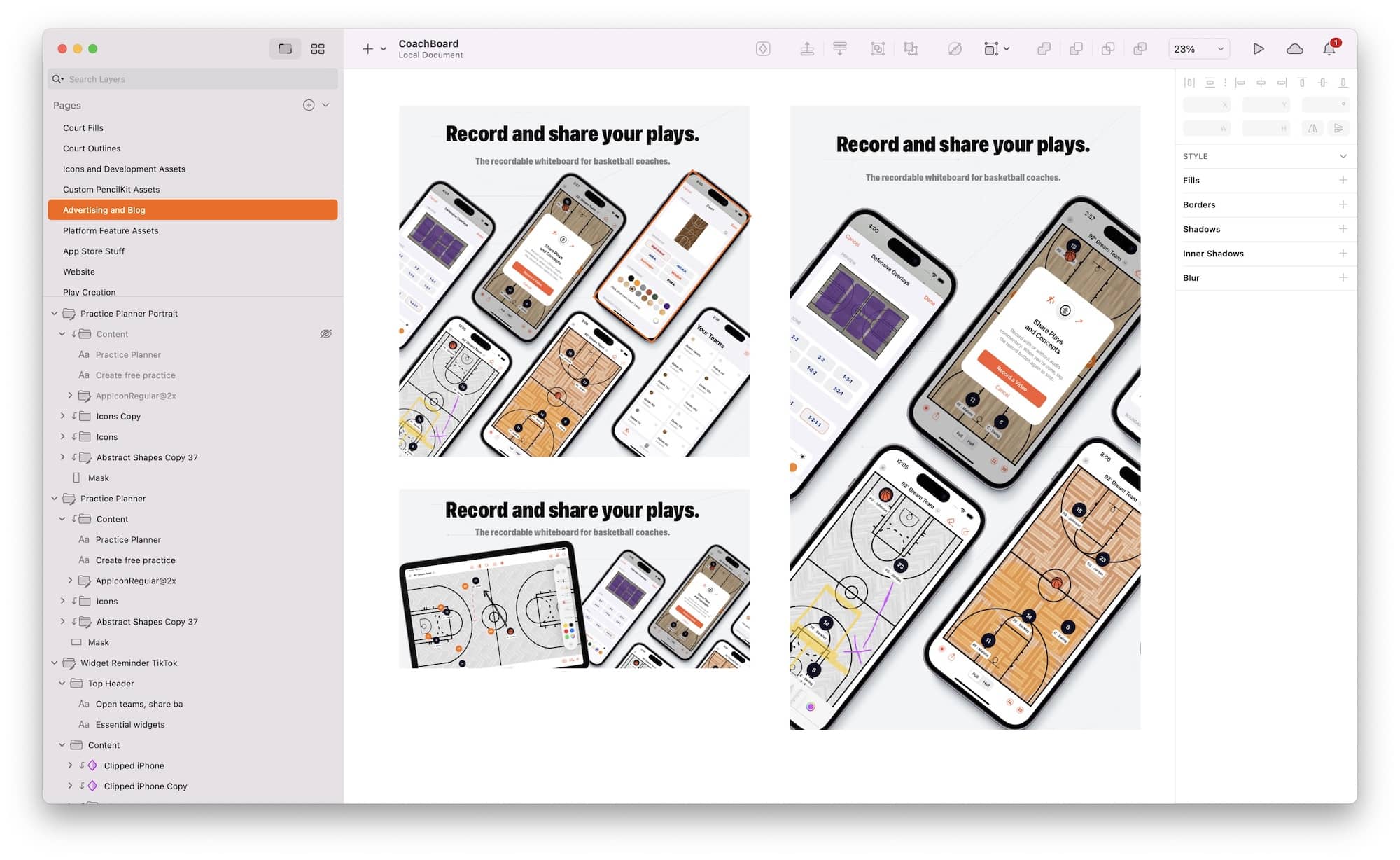
3: Side-Side Project Marketing
I love what I call “side-side project” marketing. Give a useful tool away for free. Get backlinks to it. Spread awareness. That’s exactly what I did with my Youth Basketball Practice Planner, a little web app that creates practice plans that you can customize and share.
This means that:
- People use a good tool, who are my target customers, for free.
- They like it, and they share it because they have a reason too (i.e. “Check out this practice I came up with for this week”)
- Now, they download Elite Hoops to get more.
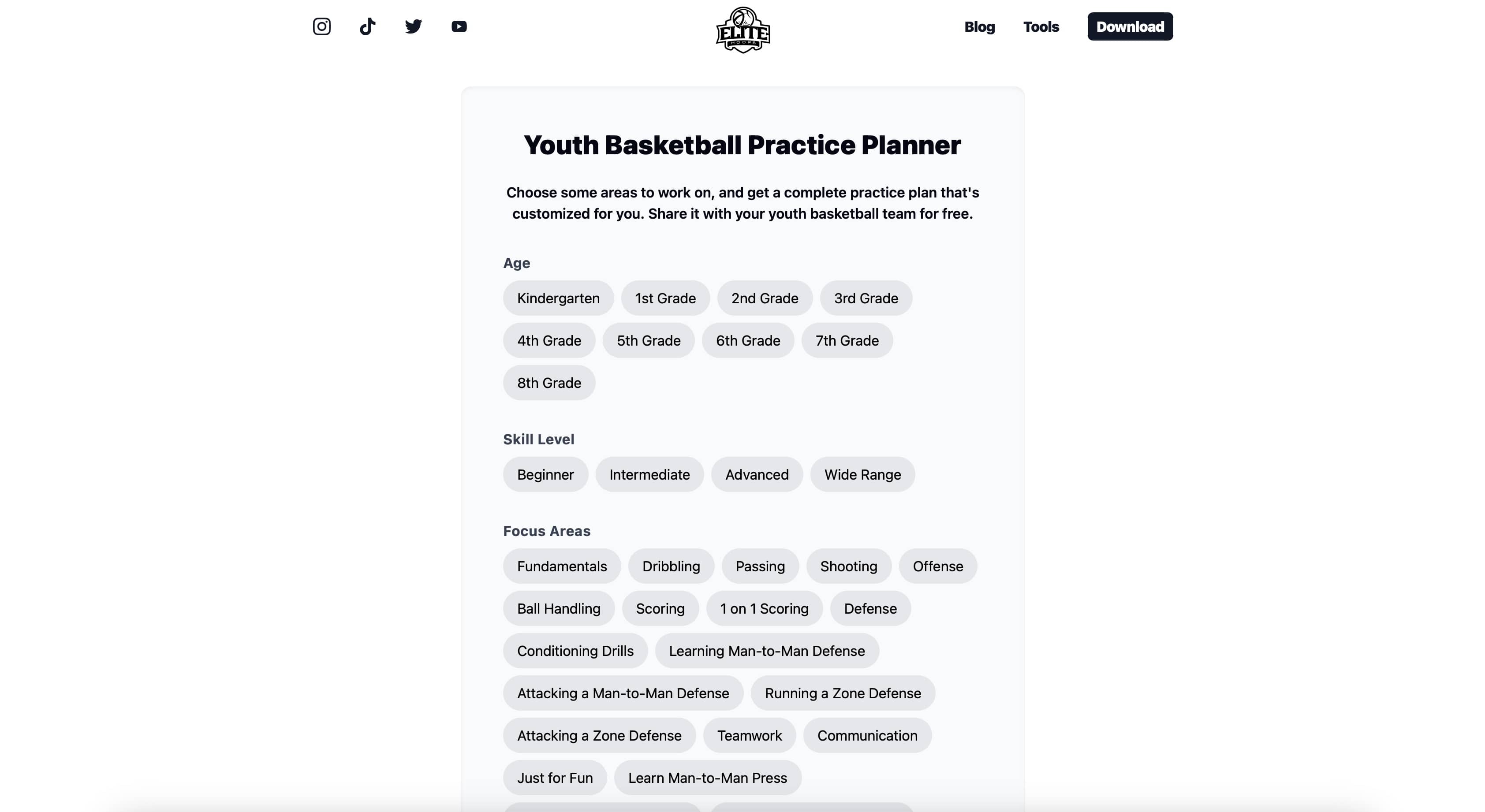
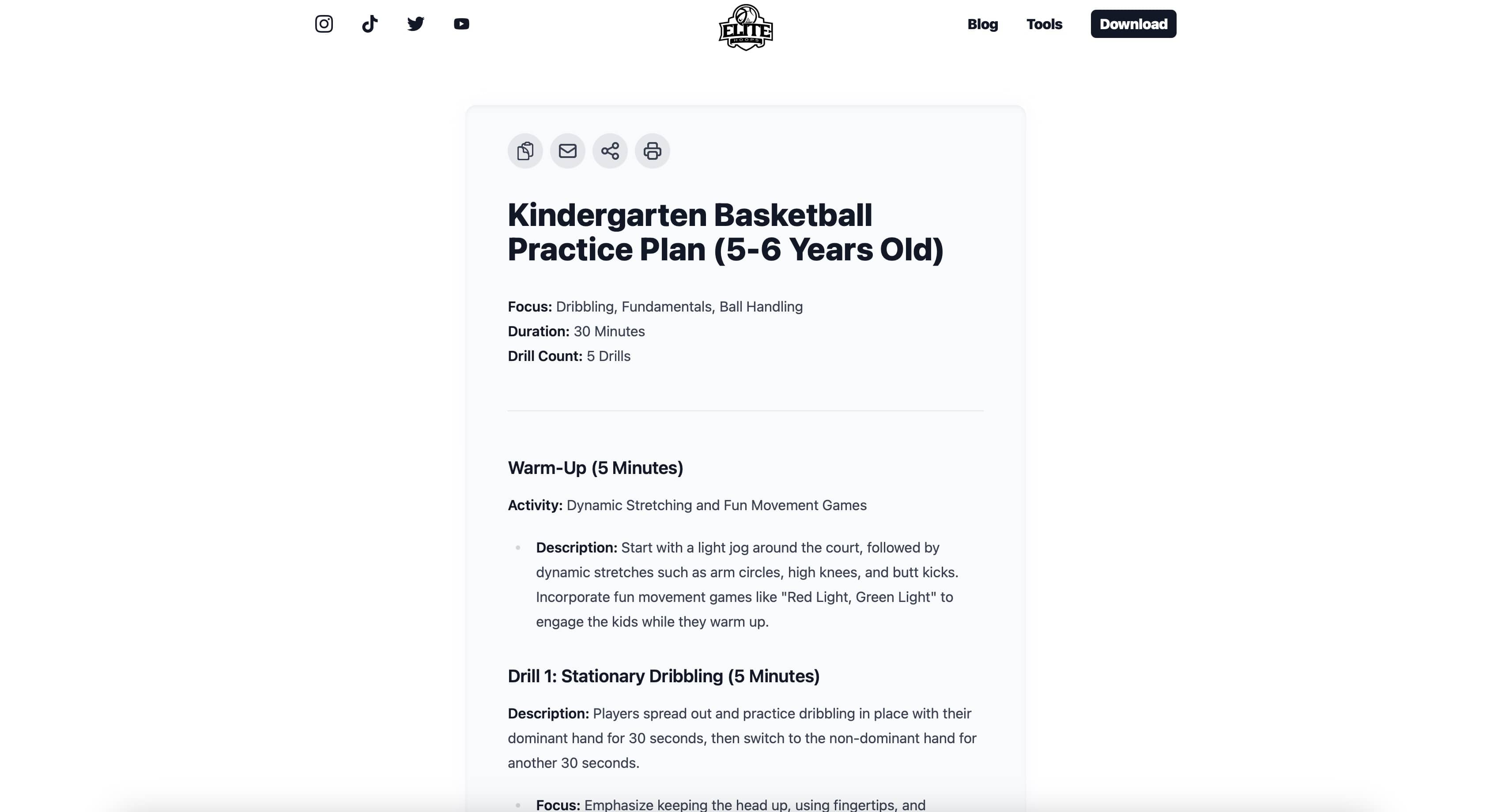
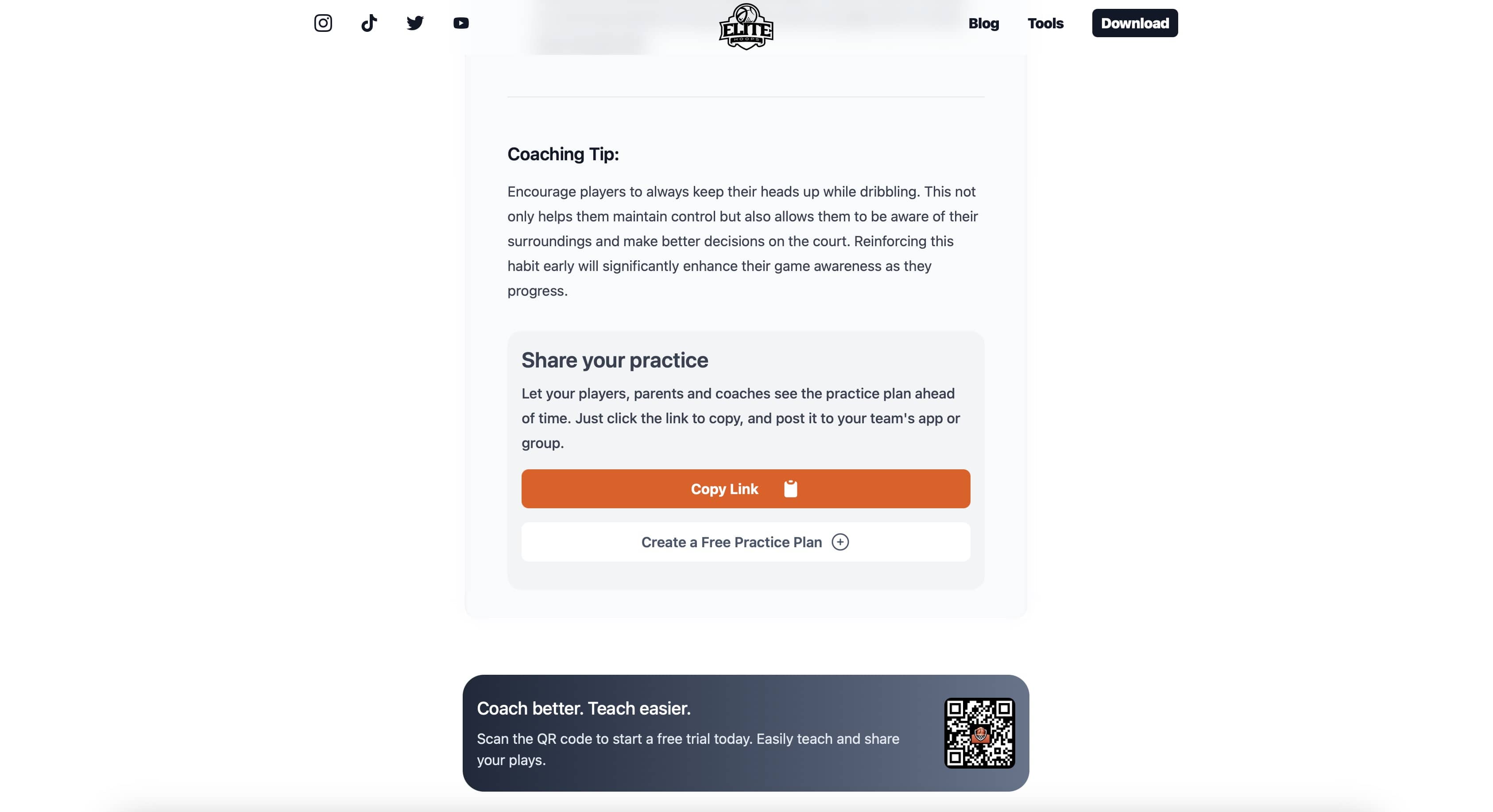
3 Thoughts on Being a Big Boi App
Nuggets on how to treat an app like the business you may want to grow it to eventually.
4: Use Mixpanel
Analytics and tracking can be done “the righteous indie way” easily. You don’t need personal information - a random identifier that shows you how people use your app is critical, and you can do it all for free with Mixpanel.
This is how I know that half of my users are youth basketball coaches, which is why I built the tool above:
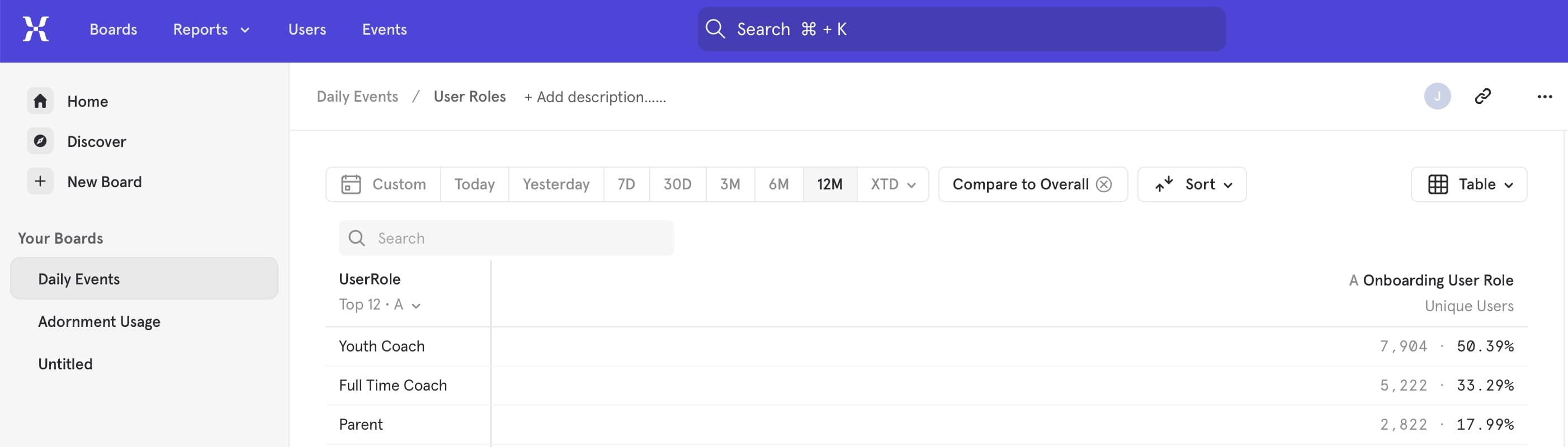
And the code for it is trivial:
struct Telemetry {
static func initializeClient() {
Mixpanel.initialize(token: "YOUR_TOKEN", trackAutomaticEvents: true)
}
static func createdDemoCourt() {
guard !Device.current.isSimulator else { return }
Mixpanel.mainInstance().track(event: "Demo Court Created")
}
// etc etc....
}
5: Use Plausible
Along the same lines, Plausible is wonderful for privacy-based web analytics. Once I double-down on some SEO plays, this is how I will know if what I’m trying is working.
6: Use Supabase for In-App Feedback
Slot in Supabase for easy feature requests. Again, they have a very nice Swift package (an indie started it, and they hired him on full time) and it takes minutes to get going. I wrote a post on how to get this up and running here.
I can’t tell you how valuable the stuff in here is:
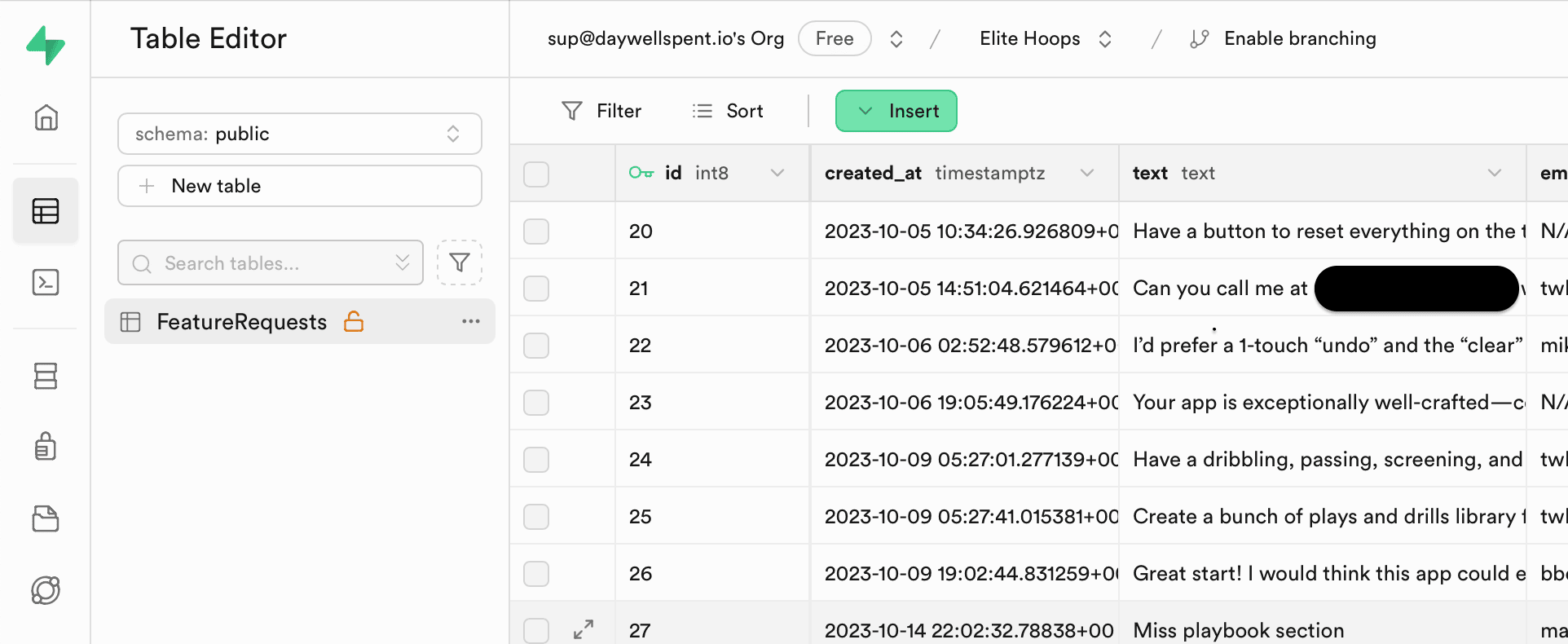
3 Indie Mindset Vitamin Shots
On ways to motivate your mindset in the indie world.
7: Think Small, Achievable Chunks
If I thought to myself, “I want to get to over 800 paying customers in a year” I probably would’ve stressed out. But, by breaking down that goal to mini ones, it was much more doable, “Get 100”, “Okay, maybe we can crack 200 in a few months”, etc.
8: Bake In Marketing
Indies don’t market enough. And, we can’t really learn much from the “winners” who had something go viral for whatever reason because that’s unlikely to happen to you or me. So, spend a whole day of your indie work on marketing.
I have this reminder weekly, #ItAintMuchButItsSomething:
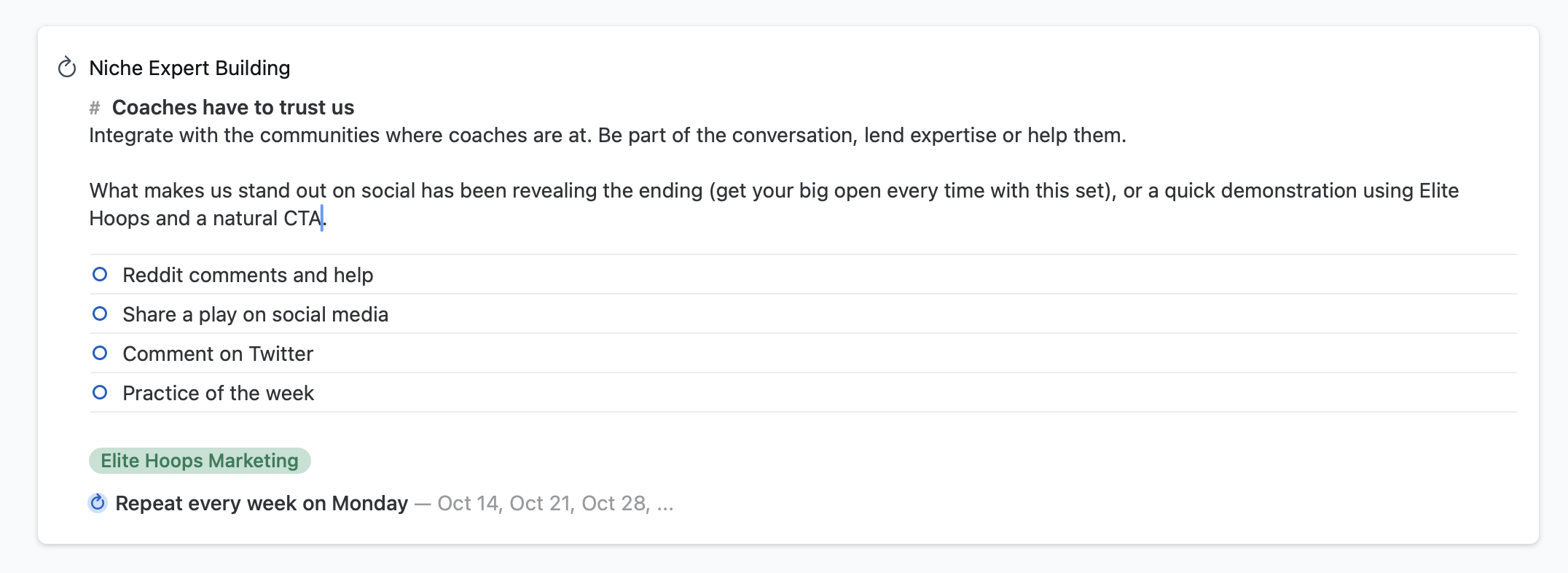
The above is the bare minimum, but while I’m in build mode (more on that below) it’s still going to help spread the word.
9: …And Figure Out If You’re in Build Mode or Marketing Mode
Are you building core functionality that’s going to get you to the next step? If you’re not, then you should really spend a lot more effort on marketing.
All of these stories going around about the Gen Z folks making millions on the App Store? They market so well. You can build an amazing thing that nobody knows about, and at the end of the day it will still be an amazing thing that nobody knows about.
3 Overall Lessons
Stuff I’ve picked up along the way.
10: Wow, Copywriting Matters
This is an area I’ve tried to grow and learn more about over the last year. And yeah, chatGPT really shines here for me. Consider selling the original iPod:
“100 GB of storage.” or “1,000s of songs in your pocket.”
One is a technical fact, the other tells a story. I’m prone to spitting out technical facts and not telling a basketball coach why something is great. So, often I’ll do a prompt this like: “I’m working on X feature, it does Y. My attempt at marketing copy was Z. Assume you are an expert in marketing in the basketball and tech space, how would you write about this feature?”
11: Staying Focused is the Hardest Part for Me
You’ll just see story after story after story about how some app, tool or gold rush is happening — and how much money is in it. And, while there is definitely value in jumping on the moment, I find that for me it’s super difficult to stay focused on Elite Hoops.
My MRR is peanuts to a lot of people, and a quite a bit to others. There’s always a spectrum, so I’ve opted to not really chase other opportunities and stay focused on growing Elite Hoops.
12: Have a Roadmap!
Seriously, it sounds obvious but I used to be bad at this! Now, I have a clear picture of where I want to go, and how I will get there. I know I’m in the aforementioned “build” mode because of the feedback I’ve received from paying users. Once I have that in, I’ll shift more towards marketing mode (but, again, don’t stop doing marketing ever!).
Wrapping Up
And that’s it! I hope this time next year, Elite Hoops has continued to grow and I’ll have more (hopefully helpful) things to share. Let’s build, baby!
Until next time ✌️
-
And, I don’t even use their SDK. You can run install ads without it, you just won’t get accurate results in terms of being able to say ‘This install was definitely from Facebook or Instagram’, but I have enough data to have a good idea of what I get from it. ↩